Introduction to Axios HTTP Client
If you want to create web applications that communicate smoothly with web servers and REST APIs, you need a reliable and easy-to-use tool for making HTTP requests and handling responses. Axios HTTP Client Library is a JavaScript library a powerful tool that does exactly that. It has become very popular among front-end developers for its simplicity, flexibility, and rich features. In this blog post, we’ll explore what Axios is and why it is a great choice for managing HTTP requests in web applications.
What is Axios HTTP Client?
Axios is an HTTP client for the browser and Node.js that uses Promises to handle asynchronous operations. It makes it very convenient to make HTTP requests to web services and REST APIs. Unlike the native fetch API, Axios has many built-in features that make working with HTTP requests and responses much easier.
Key Features of Axios
Promise-based
Axios uses Promises to handle asynchronous operations, making it more readable and manageable, especially when dealing with multiple requests or chaining actions.
Browser and Node.js support
You can use Axios both in the browser and in Node.js environments, which makes it versatile for various types of projects.
Concise syntax
Axios offers a straightforward syntax for making requests and handling responses. It encapsulates the complexities of creating and configuring requests, handling headers, and managing response data.
Request and response interception
You can intercept requests and responses to modify or log data before they are sent or processed. This is particularly useful for adding authentication tokens or logging network activity.
Automatic JSON parsing
Axios automatically parses JSON responses, simplifying the process of extracting data from responses.
Error handling
Axios provides built-in error handling that covers network errors, timeouts, and HTTP error status codes, making it easier to manage failures gracefully.
Cancellation
Axios allows you to cancel requests, which can be essential for managing dynamic web interfaces where users frequently change their actions.
Request and response transformation
Interceptors in Axios enable you to transform requests and responses globally, making it easier to standardize data or modify requests as needed.
Axios for all HTTP Requests
When using Axios, you can choose the appropriate method based on the action you want to perform, such as retrieving data, creating records, updating data, or deleting resources.
- GET: Retrieve data from the server without modifying it. This is the most common method used for fetching resources.
- POST: Send data to the server to create a new resource. This method is often used for submitting form data or creating records.
- PUT: Send data to the server to update or replace an existing resource. It typically requires sending the entire updated resource.
- PATCH: Send data to the server to partially update an existing resource. Unlike PUT, it only requires sending the changes.
- DELETE: Request the server to remove a resource.
- HEAD: Similar to GET, but only requests the headers of the response, not the actual data. Useful for checking resource status.
- OPTIONS: Retrieve information about the communication options available for the target resource.
- CONNECT: Establish a network connection to a resource, often used to set up a network tunnel.
- TRACE: Echoes the received request back to the client, providing a way to see what intermediate servers received and how they interpreted the request.
Start Using Axios
Installation
Begin by installing Axios as a dependency in your React project
npm install axios
//or
yarn add axios
Import Axios
Import Axios into the component where you want to perform HTTP requests.
import axios from 'axios';
Syntax of Axios
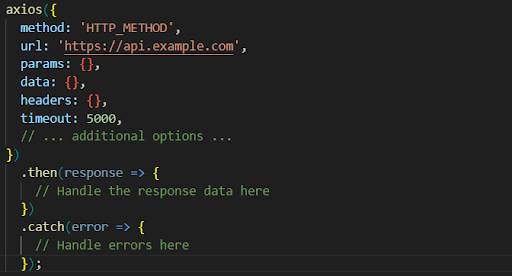
Or you can your Axios short hand syntax,
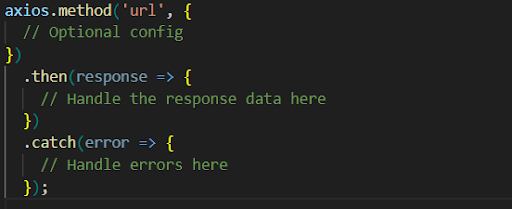
Options to Configure Request with Axios
Axios provides a wide range of options that you can include in a request configuration object. Here are some of the common options you might want to include:
- method: Specifies the HTTP method for the request (e.g., ‘GET’, ‘POST’, ‘PUT’, ‘DELETE’).
- url: The URL to which the request is sent.
- params: An object containing URL parameters to be appended to the URL for a GET request.
- data: The data to be sent as the request body for POST, PUT, and PATCH requests.
- headers: An object containing custom headers to be included in the request.
- timeout: The maximum time, in milliseconds, the request is allowed to take before being canceled.
- auth: An object containing credentials for HTTP Basic Authentication.
More Options
There are many other options you can explore as follows,
- cancelToken: A cancel token that can be used to cancel the request.
- responseType: The type of data to expect in the response (e.g., ‘json’, ‘text’, ‘blob’, ‘stream’).
- maxRedirects: The maximum number of redirects to follow.
- onUploadProgress: A callback function to track the upload progress of data.
- onDownloadProgress: A callback function to track the download progress of the response.
- validateStatus: A custom function to determine if a response’s status code is considered successful.
- maxContentLength: The maximum allowed size for the response content in bytes.
- transformRequest: An array of functions to transform the request data before sending.
- transformResponse: An array of functions to transform the response data before resolving.
- proxy: The URL of a proxy server to use for the request.
- withCredentials: Use this option to make cross-site Access-Control requests with credentials such as cookies or authorization headers.
These are just a few of the many options available in Axios. Depending on your specific use case and requirements, you can choose which options to include in your request configuration object to tailor the behavior of the request and the response handling.
For a comprehensive list of all available options and their descriptions, you can refer to the official Axios documentation: Request Config and Response Schema.
HTTP Request Example with Axios
GET Request
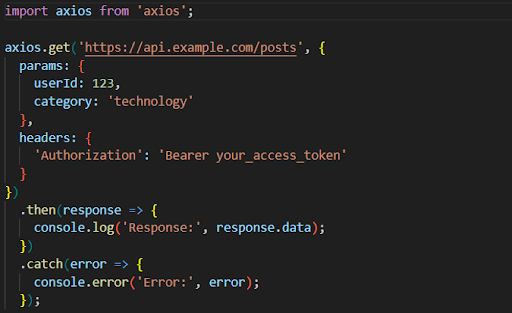
In this example:
- We are making a GET request to ‘https://api.example.com/posts’ to fetch posts.
- Append query parameters (userId and category) to the URL using the params object.
- The headers object includes an authorization header with an access token.
- In the .then() block, we handle the successful response by logging the response data.
- In the .catch() block, we handle errors by logging the error information.
Make sure to replace ‘https://api.example.com’ with the actual API endpoint you’re working with, and adjust the params object to match the query parameters expected by the API. Also, replace ‘Bearer your_access_token’ with an appropriate authorization token if your API requires one.
POST Request
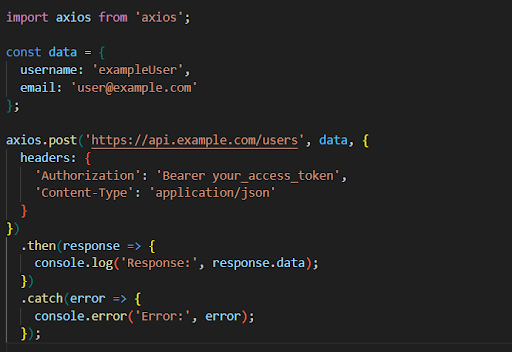
In this example:
- We are making a POST request to ‘https://api.example.com/users’ to create a new user.
- Send the user information in the request body using the data object.
- The headers object includes an authorization header with an access token and specifies that the content type of the request is JSON.
- In the .then() block, we handle the successful response by logging the response data.
- In the .catch() block, we handle errors by logging the error information.
Remember to replace ‘https://api.example.com’ with the actual API endpoint you’re targeting and adjust the data object to match the data structure expected by the API. Additionally, replace ‘Bearer your_access_token’ with a valid authorization token if required by your API.
Same way you can modify the Axios general syntax for other HTTP requests.
Final Thought
Axios can be a great asset for your React projects, whether they are small or large, simple or complex. It can help you streamline your workflow and improve your application’s performance. With its easy-to-use syntax, rich features, and smooth integration, Axios is a powerful tool for any React developer.
You can refer to the official Axios documentation for a comprehensive guide and detailed information.