In the world of web development, creating complex applications often involves managing the application’s state. State management libraries like Redux have proven instrumental in handling state in a predictable and maintainable manner. However, setting up and configuring Redux can be quite daunting, leading to boilerplate code and decreased development efficiency. This is where React Toolkit for state management come to rescue.
Redux Toolkit: Simplifying State Management
Redux Toolkit is a set of utilities that simplifies the process of working with Redux by providing developers with a streamlined way to write Redux code. It’s designed to address common challenges faced when setting up and managing a Redux store, while also promoting best practices and reducing boilerplate code.
Key Features of Redux Toolkit
Redux Store Setup Simplification
Creating a Redux store can be a cumbersome task. Redux Toolkit’s configureStore function abstracts away the store setup process, automatically configuring the store with essential middleware and devtools extension integration.
Reducers Made Easy with createSlice
Reducers are at the core of Redux, but they often lead to verbose code. Redux Toolkit’s createSlice function lets you define a reducer along with its associated actions in a concise manner, reducing the need for writing switch cases and action creators.
Immutability Made Simple
Immutability is crucial for maintaining a predictable state. Redux Toolkit internally uses the immer library, which enables you to write mutable code that is turned into immutable updates, making state updates more intuitive and straightforward.
Built-in Thunk Middleware
Asynchronous actions are a common requirement in modern applications. Redux Toolkit includes the createAsyncThunk function, which simplifies the process of handling asynchronous operations and side effects, such as API calls.
Performance Optimizations
Redux Toolkit comes with built-in optimizations such as memoized selectors, which efficiently compute derived state and reduce unnecessary re-renders.
Dev-friendly Development
Redux Toolkit encourages good development practices by providing a clean and structured way to manage state. The built-in Redux DevTools Extension integration helps with debugging and time-traveling through state changes.
Getting Started with Redux Toolkit
Setting Up Your Project
Assuming you have a basic React project set up, make sure you have Redux and Redux Toolkit installed:
npm install @reduxjs/toolkit react-redux
Now lets start with basic counter application using Redux Toolkit and React. We’ll begin by configuring a Redux store with a “counter” slice that holds the count state, defining its initial value and includes actions to increment and decrement it. Then, we’ll create React components that utilize the Redux store and access the state using useSelector
and update it via useDispatch
. The counter value will be displayed, and dedicated buttons will trigger the corresponding actions to either increase or decrease the count.
Create the Redux Store
In your src
folder, create a new file named store.js
:
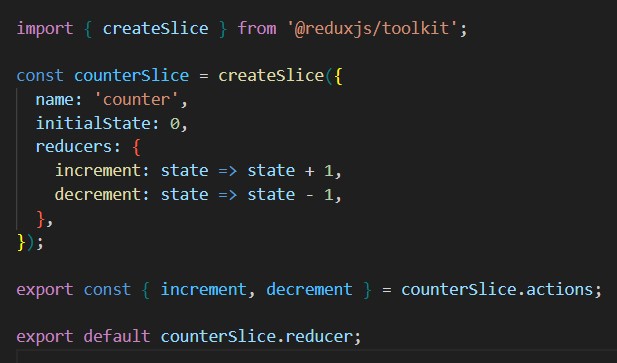
Creating the Redux Slice
A “slice” in Redux Toolkit is a way to organize and encapsulate reducer logic. Let’s create a slice for our counter.
Create a file named counterSlice.js
:
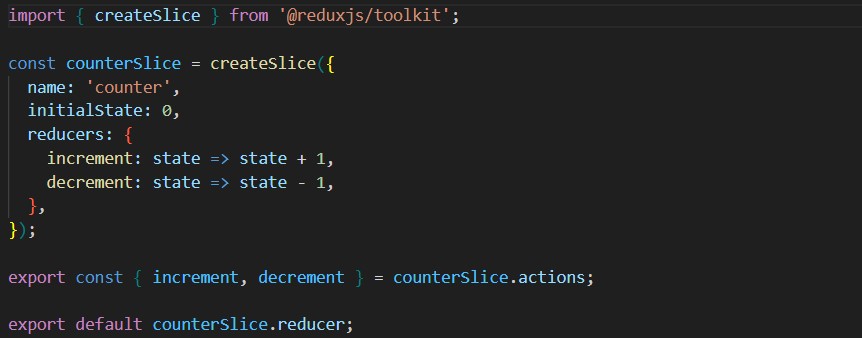
Creating the Counter Component
Create a file named Counter.js
in the src
folder and add the following code:
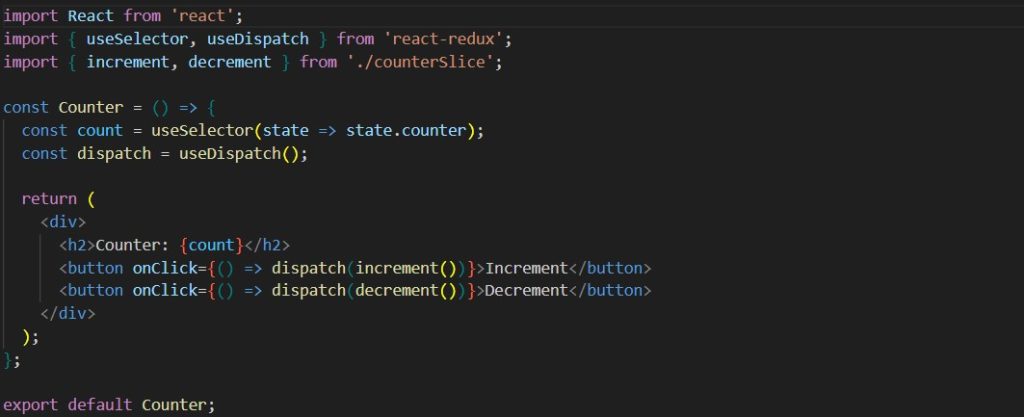
Updating the Main App Component
Open the src/App.js
file and update it to include the Counter
component:
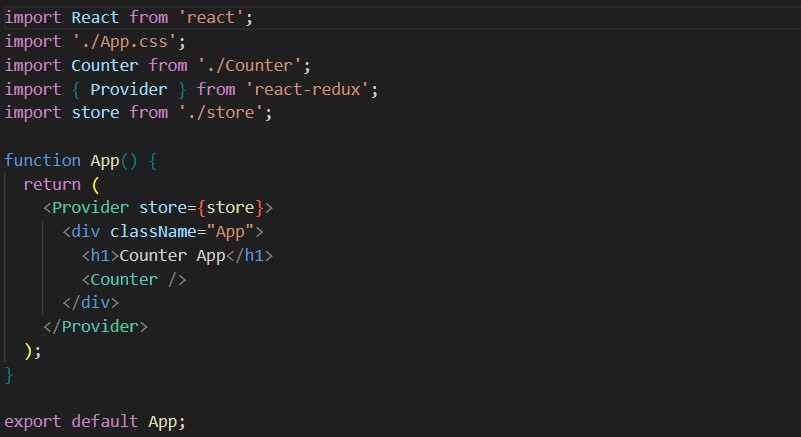
At last
Redux Toolkit is a game-changer in state management of an application, simplifying the complexities of Redux while promoting best practices and improved development efficiency. By leveraging Redux Toolkit’s features like createSlice, createAsyncThunk, and the built-in store configuration, developers can focus more on building robust applications and less on managing intricate state updates. Whether you’re new to Redux or a seasoned developer, incorporating Redux Toolkit into your workflow can lead to cleaner, more maintainable code and a smoother development experience overall.
Hope you find the post informative, go to our Blogs page for such posts on React concepts and more.
For more about Redux Toolkit visit the official page at https://redux-toolkit.js.org/.